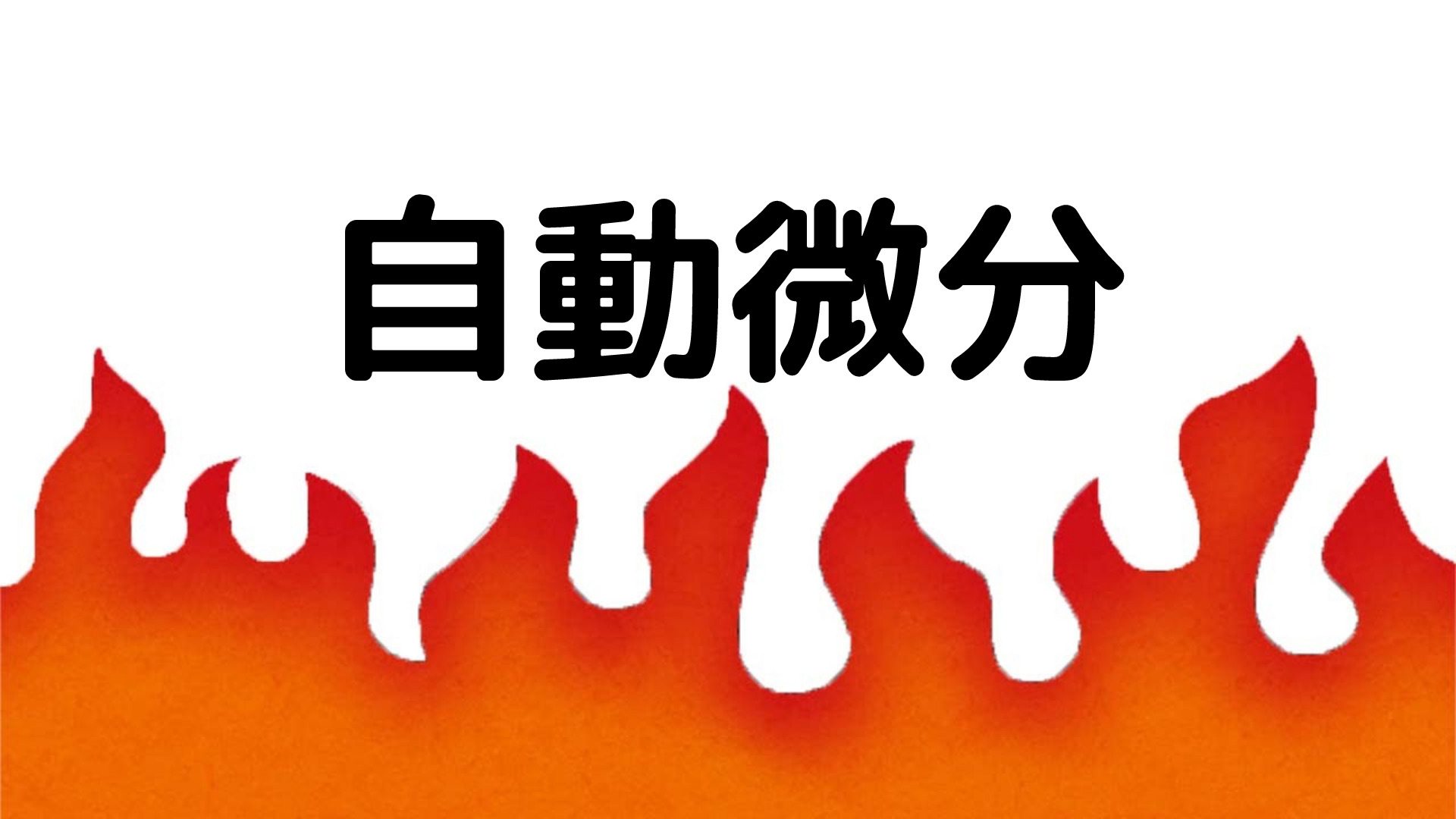
【pytorchでニューラルネットワーク#2】自動微分(backward)
記事の目的
pytorchでニューラルネットワークを実装する上で必要になる自動微分について実装していきます。ここにある全てのコードは、コピペで再現することが可能です。
目次
1 ライブラリ
# In[1] import torch from torch import nn torch.manual_seed(1)
2 線形変換と自動微分
# In[2] x = torch.tensor(10) w = torch.tensor(3, requires_grad=True, dtype=torch.float64) b = torch.tensor(5, requires_grad=True, dtype=torch.float64) # In[3] def linear(x,w,b): y = w*x+b return y # In[4] y = linear(x,w,b) y # In[5] y.backward() # In[6] w.grad, b.grad
3 線形変換とMSE
# In[7] x = torch.tensor(10) w = torch.tensor(3, requires_grad=True, dtype=torch.float64) b = torch.tensor(5, requires_grad=True, dtype=torch.float64) t = torch.tensor(32) # In[8] def MSE(y,t): L = (y-t)**2 return L # In[9] y = linear(x,w,b) L = MSE(y,t) # In[10] y, L # In[11] L.backward() # In[12] w.grad, b.grad
4 ライブラリの利用
# In[13] x = torch.tensor([1,2,3,4,5]).unsqueeze(1).float() t = torch.tensor([5,8,11,14,17]).unsqueeze(1).float() # In[14] linear = nn.Linear(1,1) MSE = nn.MSELoss() # In[15] y = linear(x) L = MSE(y,t) # In[16] L.backward() # In[17] linear.weight, linear.bias # In[18] linear.weight.grad, linear.bias.grad