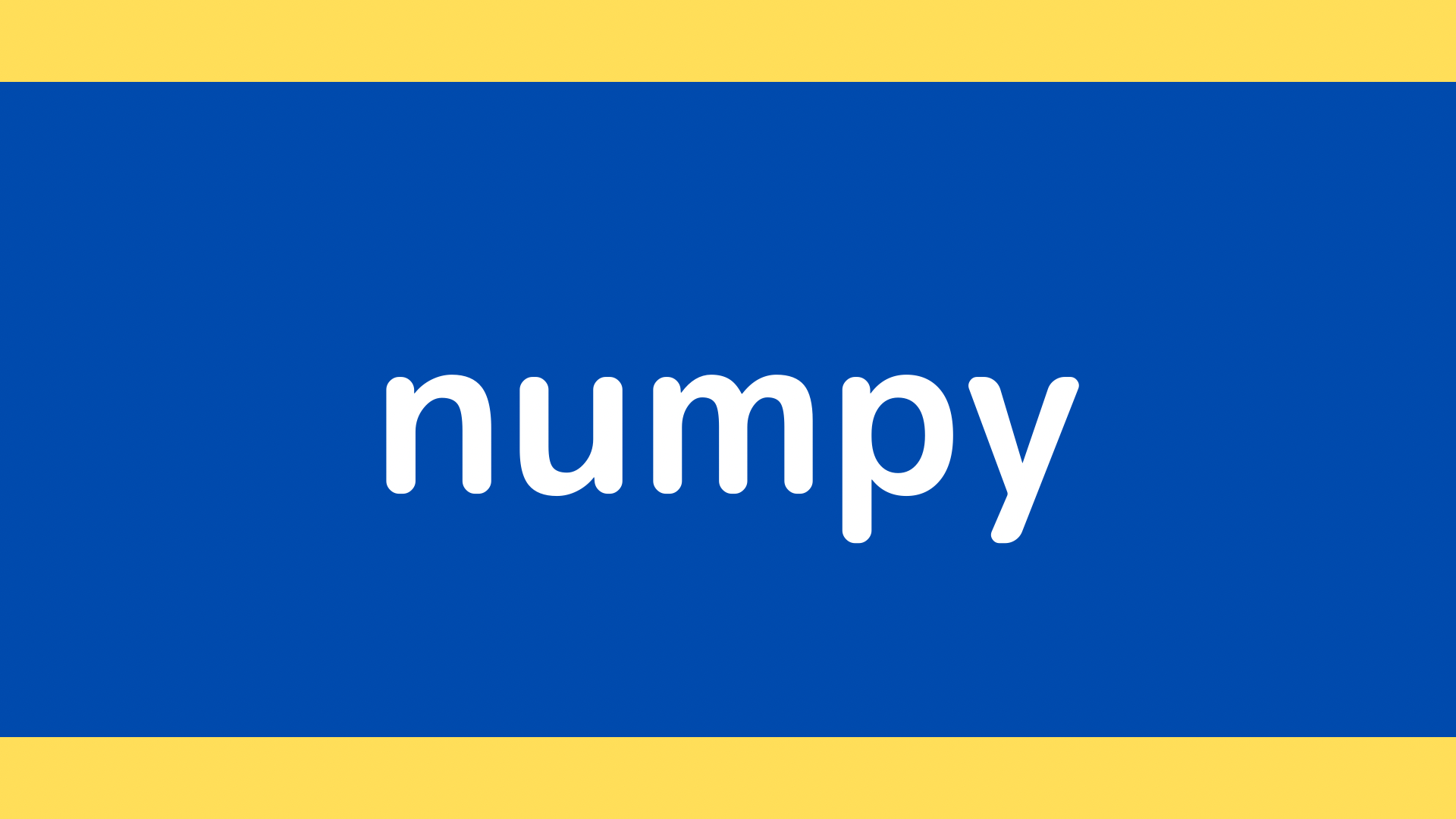
【pythonの基礎#2】データの扱い(numpy)
記事の目的
pythonの基礎である、データを扱う際に使用するnumpyについて実装していきます。ここにある全てのコードは、コピペで再現することが可能です。
目次
1 ライブラリとデータの作成
import numpy as np # 1.1 次元 x0 = np.array(1) print(x0, x0.shape) # スカラー x1 = np.array([1,2,3,4]) print(x1, x1.shape) # ベクトル x2 = np.array([[1,2],[3,4]]) print(x2, x2.shape) # 行列 # 1.2 連続データ x = np.arange(10) # 0-9 print(x) # 1.3 ランダムデータ np.random.seed(1) x = np.random.rand(2, 2) # 0-1の一様分布からの乱数発生 print(x) x = np.random.randn(2, 2) # 標準正規分布からの乱数発生 print(x) # 1.4 0と1 x = np.zeros((3,5)) # 全要素が0の行列 print(x) x = np.ones((5,4)) # 全要素が1の行列 print(x)
2 データの参照
x = np.random.randn(3,5) print(x) print(x[0,:]) # 0行目 print(x[:,3]) # 3列面 print(x[0:2,:]) # 0-1行目
3 演算
# 3.1 ベクトルの演算 x = np.array([1,2,3,4]) y = np.array([5,6,7,8]) print(x+10,x-10,x*10,x/10) # スカラーとの四則演算 print(x+y,x-y,x+y,x/y) # ベクトル同士の四則演算 print(np.dot(x,y)) # ベクトルの内積 # 3.2 行列の演算 x = np.array([[1,2],[3,4]]) y = np.array([[5,6],[7,8]]) print(x+10) # スカラーとの四則演算 print(x+y) # 行列同士の四則演算 print(np.dot(x,y)) # 行列の内積
4 特殊な関数
# 4.1 sumとmean x = np.array([[1,2],[3,4]]) print(x) print(x.sum()) # 全要素の合計 print(x.sum(axis=0), x.sum(axis=1)) # 各軸の合計 print(x.mean()) # 全要素の平均 print(x.mean(axis=0), x.mean(axis=1)) # 各軸の平均 # 4.2 reshapeとtranspose x = np.arange(30) print(x.reshape(5,6)) # 形を変形 print(x.reshape(2,3,5)) print(x.reshape(3,-1)) y = x.reshape(2, 3, 5) y.transpose(1,0,2)