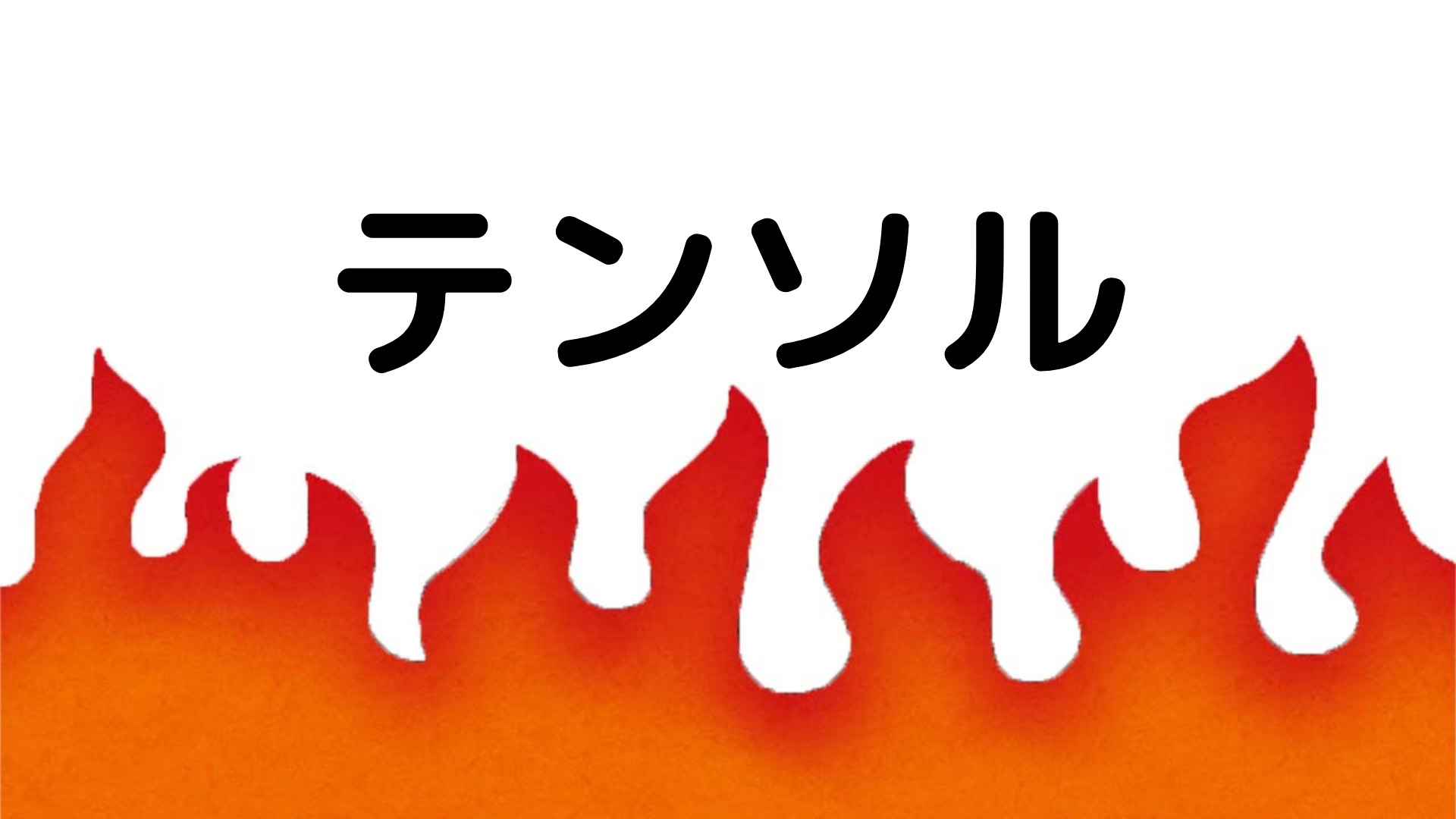
【pytorchでニューラルネットワーク#1】テンソルの基礎
記事の目的
pytorchでニューラルネットワークを実装する上で必要になるテンソルの基礎について実装していきます。ここにある全てのコードは、コピペで再現することが可能です。
目次
1 ライブラリとデータの作成
# In[1] import numpy as np import torch torch.manual_seed(1) # In[2] x0 = torch.tensor(1) print(x0.shape, x0) # In[3] x1 = torch.tensor([1,2,3,4]) print(x1.shape, x1) # In[4] x2 = torch.tensor([[1,2],[3,4]]) print(x2.shape, x2, sep="\n") # In[5] x3 = torch.tensor([[[1,2,3],[4,5,6],[7,8,9]],[[1,2,3],[4,5,6],[7,8,9]]]) print(x3.shape, x3, sep="\n") # In[6] x4 = torch.tensor([[[[1,2],[3,4]],[[1,2],[3,4]],[[1,2],[3,4]]],[[[1,2],[3,4]],[[1,2],[3,4]],[[1,2],[3,4]]]]) print(x4.shape, x4, sep="\n") # In[7] x = torch.arange(5) x # In[8] x = torch.arange(1,5) x # In[9] x = torch.arange(1,10,2) x # In[10] x = torch.rand(2,2) x # In[11] x = torch.randn(3,3) x # In[12] x = torch.normal(5, 1, size=(6,)) print(x.shape, x)
2 データの変形
# In[13] y = x.view(2,3) print(y.shape, y, sep="\n") # In[14] y = x.unsqueeze(1) print(y.shape, y, sep="\n")
3 データの参照
# In[15] x = torch.randn(3,4) x # In[16] x[0,] # In[17] x[:,2] # In[18] x[:,0:2]
4 numpyとテンソル
# In[19] x = np.arange(10) x # In[20] y = torch.tensor(x) y # In[21] y = torch.from_numpy(x) y # In[22] y.numpy()